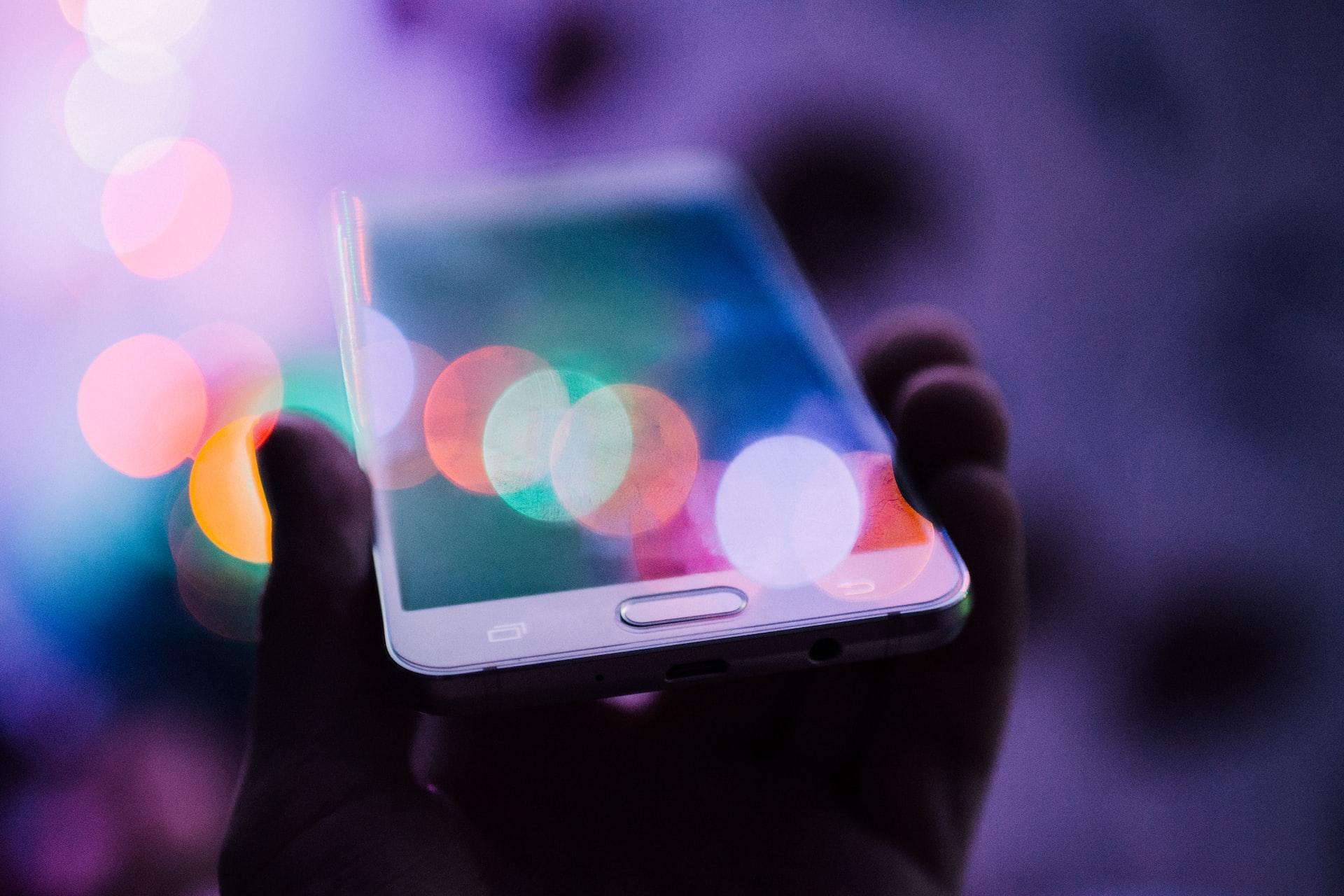
🧪 How to set up Jest & React Native Testing Library in your Expo App! 💥
If you've used React Testing Library in a regular React project before then the React Native version will already seem familiar, however getting everything setup and configured is usually the trickiest part!
What we'll be using:
Prerequisites
- A React Native Expo project.
Create a new one if you don't have one to work on already. See the Expo docs on creating a new project)
Installing React Native Testing Library.
Go ahead and install React Native Testing Library. I'm using npx expo install since I'm using Expo:
npx expo install @testing-library/react-native -- --save-dev
Installing react-test-renderer
We'll also need to install react-test-renderer, as React Native Testing Library uses it as a dependency.
IMPORTANT: Before you install it, look in your package.json and check what version of react you are using. You need to install the same version of react-test-renderer for compatibility. For example, I'm using "react": "18.1.0" - Therefore I need to install "react-test-renderer": "18.1.0":
npx expo install react-test-renderer@18.1.0
Installing Jest
Finally, go ahead and follow the instructions to install jest here. Be sure to add the configuration to your package.json
Test Examples
//App.test.js import renderer from "react-test-renderer"; import { render } from "@testing-library/react-native"; describe("<App />", () => { it("has 1 child", () => { const tree = renderer.create(<App />).toJSON(); expect(tree.children.length).toBe(1); }); it("renders correctly", () => { const tree = renderer.create(<App />).toJSON(); expect(tree).toMatchSnapshot(); }); it("renders Hello World message on the home page", async () => { render(<App />); expect(screen.getByText("Hello, World!")).toBeDefined() }); }); // NOTE: You can use either: // renderer.create(<App />) from "react-test-renderer" // or render(<App />) from "@testing-library/react-native"
The above tests are based on a super simple app with only one child. If you have a more complex app with additional libraries, then there may be more setup required, and I will go into these scenarios in more detail in future articles.
Please feel free to comment anything useful or interesting you've found when setting up testing on your Expo project.
Happy testing!